I was struggling to find a way to use chatify in my laravel app, after some tweaking I managed to get it to work, I will post the code I used to get it to work with an existing app.
For the realtime chat to work you will need a Pusher account, go to channels and create a new app or click this link
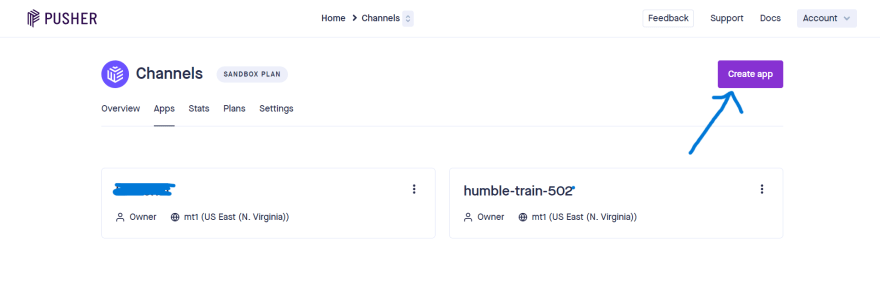
- Select your preferred frontend and backend languages to get code snippets (This can be changed later on). For this app, I selected jQuery and Laravel for the frontend and backend respectively.

- Navigate to app keys as shown and copy the values to your Laravel

- Enable client events in the Pusher application:

- Replace these values in your .env Laravel with the appropraite values you copied from the app keys online
PUSHER_APP_ID=
PUSHER_APP_KEY=
PUSHER_APP_SECRET=
PUSHER_APP_CLUSTER=
You also need to update your BROADCAST_DRIVER=pusher
I will assume you already have a laravel app up and running, you will need to install the chatify script using composer; Here is the official documentation for chatify.
Run the following command to install Chatify:
$ composer require munafio/chatify
Configure and publish Chatify assets, migrations and storage symlink:
$ php artisan chatify:install
Run migrations:
$ php artisan migrate
Ok now chatify is installed you will need to make some changes to use it in your own app views, this is what mine looks like after the edits:
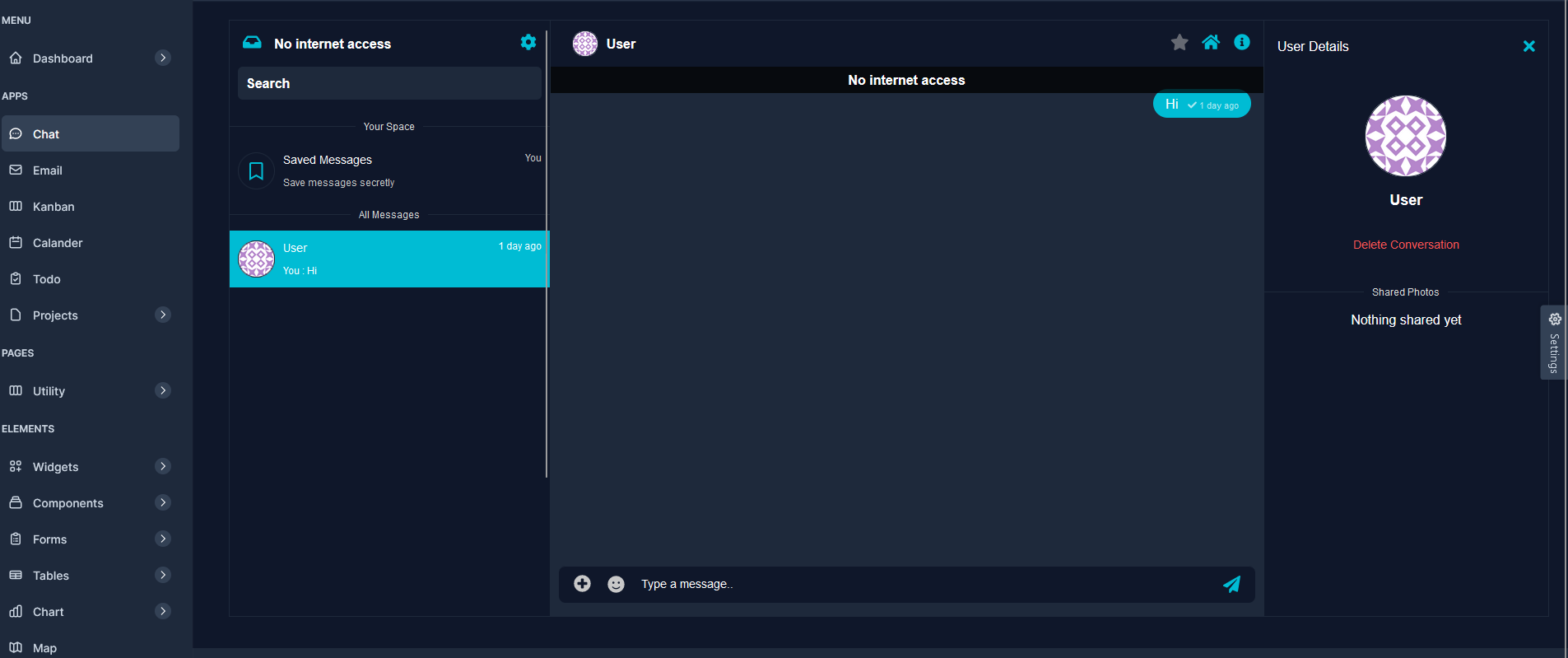
The file structure for chatify is views/vendor/Chatify/layouts and views/vendor/Chatify/pages, we will need to modify files in both of these folders.
To get Chatify to display in your blade you will need to use:
@include('vendor.Chatify.pages.app')
In the views/vendor/Chatify/pages/app.blade you will need to add your layout tags at the top and bottom of the page like this:
<x-app-layout>
<div class="space-y-8">
<div class="flex lg:space-x-5 chat-height overflow-hidden relative rtl:space-x-reverse">
@include('Chatify::layouts.headLinks')
<div class="messenger">
{{-- ----------------------Users/Groups lists side---------------------- --}}
<div class="messenger-listView {{ !!Auth::user()->id ? 'conversation-active' : '' }}">
{{-- Header and search bar --}}
<div class="m-header">
<nav>
<a href="#"><i class="fas fa-inbox"></i> <span
class="messenger-headTitle">MESSAGES</span> </a>
{{-- header buttons --}}
<nav class="m-header-right">
<a href="#"><i class="fas fa-cog settings-btn"></i></a>
<a href="#" class="listView-x"><i class="fas fa-times"></i></a>
</nav>
</nav>
{{-- Search input --}}
<input type="text" class="messenger-search" placeholder="Search" />
{{-- Tabs --}}
{{-- <div class="messenger-listView-tabs">
<a href="#" class="active-tab" data-view="users">
<span class="far fa-user"></span> Contacts</a>
</div> --}}
</div>
{{-- tabs and lists --}}
<div class="m-body contacts-container">
{{-- Lists [Users/Group] --}}
{{-- ---------------- [ User Tab ] ---------------- --}}
<div class="show messenger-tab users-tab app-scroll" data-view="users">
{{-- Favorites --}}
<div class="favorites-section">
<p class="messenger-title"><span>Favorites</span></p>
<div class="messenger-favorites app-scroll-hidden"></div>
</div>
{{-- Saved Messages --}}
<p class="messenger-title"><span>Your Space</span></p>
{!! view('Chatify::layouts.listItem', ['get' => 'saved']) !!}
{{-- Contact --}}
<p class="messenger-title"><span>All Messages</span></p>
<div class="listOfContacts"
style="width: 100%;height: calc(100% - 272px);position: relative;"></div>
</div>
{{-- ---------------- [ Search Tab ] ---------------- --}}
<div class="messenger-tab search-tab app-scroll" data-view="search">
{{-- items --}}
<p class="messenger-title"><span>Search</span></p>
<div class="search-records">
<p class="message-hint center-el"><span>Type to search..</span></p>
</div>
</div>
</div>
</div>
{{-- ----------------------Messaging side---------------------- --}}
<div class="messenger-messagingView">
{{-- header title [conversation name] amd buttons --}}
<div class="m-header m-header-messaging">
<nav class="chatify-d-flex chatify-justify-content-between chatify-align-items-center">
{{-- header back button, avatar and user name --}}
<div class="chatify-d-flex chatify-justify-content-between chatify-align-items-center">
<a href="#" class="show-listView"><i class="fas fa-arrow-left"></i></a>
<div class="avatar av-s header-avatar"
style="margin: 0px 10px; margin-top: -5px; margin-bottom: -5px;">
</div>
<a href="#" class="user-name">{{ config('chatify.name') }}</a>
</div>
{{-- header buttons --}}
<nav class="m-header-right">
<a href="#" class="add-to-favorite"><i class="fas fa-star"></i></a>
<a href="/"><i class="fas fa-home"></i></a>
<a href="#" class="show-infoSide"><i class="fas fa-info-circle"></i></a>
</nav>
</nav>
{{-- Internet connection --}}
<div class="internet-connection">
<span class="ic-connected">Connected</span>
<span class="ic-connecting">Connecting...</span>
<span class="ic-noInternet">No internet access</span>
</div>
</div>
{{-- Messaging area --}}
<div class="m-body messages-container app-scroll">
<div class="messages">
<p class="message-hint center-el"><span>Please select a chat to start messaging</span></p>
</div>
{{-- Typing indicator --}}
<div class="typing-indicator">
<div class="message-card typing">
<div class="message">
<span class="typing-dots">
<span class="dot dot-1"></span>
<span class="dot dot-2"></span>
<span class="dot dot-3"></span>
</span>
</div>
</div>
</div>
</div>
{{-- Send Message Form --}}
@include('Chatify::layouts.sendForm')
</div>
{{-- ---------------------- Info side ---------------------- --}}
<div class="messenger-infoView app-scroll">
{{-- nav actions --}}
<nav>
<p>User Details</p>
<a href="#"><i class="fas fa-times"></i></a>
</nav>
{!! view('Chatify::layouts.info')->render() !!}
</div>
</div>
</div>
</div>
</x-app-layout>
@include('Chatify::layouts.modals')
@include('Chatify::layouts.footerLinks')
For the file edits you need to update the views/vendor/Chatify/layouts/headLinks.blade with:
<title>{{ config('chatify.name') }}</title>
{{-- Meta tags --}}
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="id" content="{{ Auth::user()->id }}">
<meta name="messenger-color" content="{{ Auth::user()->messenger_color }}">
<meta name="messenger-theme" content="{{ Auth::user()->dark_mode }}">
<meta name="csrf-token" content="{{ csrf_token() }}">
<meta name="url" content="{{ url('').'/'.config('chatify.routes.prefix') }}" data-user="{{ Auth::user()->id }}">
{{-- scripts --}}
<script
src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="{{ asset('js/chatify/font.awesome.min.js') }}"></script>
<script src="{{ asset('js/chatify/autosize.js') }}"></script>
<script src='https://unpkg.com/[email protected]/nprogress.js'></script>
{{-- styles --}}
<link rel='stylesheet' href='https://unpkg.com/[email protected]/nprogress.css'/>
<link href="{{ asset('css/chatify/style.css') }}" rel="stylesheet" />
<link href="{{ asset('css/chatify/'. (Auth::user()->dark_mode > 0 ? 'dark' : 'light').'.mode.css') }}" rel="stylesheet" />
{{-- Setting messenger primary color to css --}}
<style>
:root {
--primary-color: {{ Auth::user()->messenger_color }};
}
</style>
And the views/vendor/Chatify/layouts and views/vendor/Chatify/pages/app.blade with:
<div class="messenger">
{{-- ----------------------Users/Groups lists side---------------------- --}}
<div class="messenger-listView {{ !!Auth::user()->id ? 'conversation-active' : '' }}">
{{-- Header and search bar --}}
You need to add this line to the views/vendor/Chatify/layouts/modal.blade
<label class="app-btn a-btn-primary update"
style="background-color:{{ Auth::user()->messenger_color }}">
If you open your chat view you should now see the chatify screen displayed, one more change in the config/chatify file change the 'chat' to whatever your view is: 'prefix' => env('CHATIFY_ROUTES_PREFIX', 'chat'),
Thasts it I hoped you found this useful, if chatify is not running in realtime, check your settings and clear any cache using:
php artisan cache:clear and php artisan view:clear
or php artisan optimize:clear
Have fun!